- Published on
Understanding Redux and Redux Toolkit: A Comprehensive Guide
- Authors
- Name
- Shaheer Mansoor
- @Twitter/ShaheerMansoor2
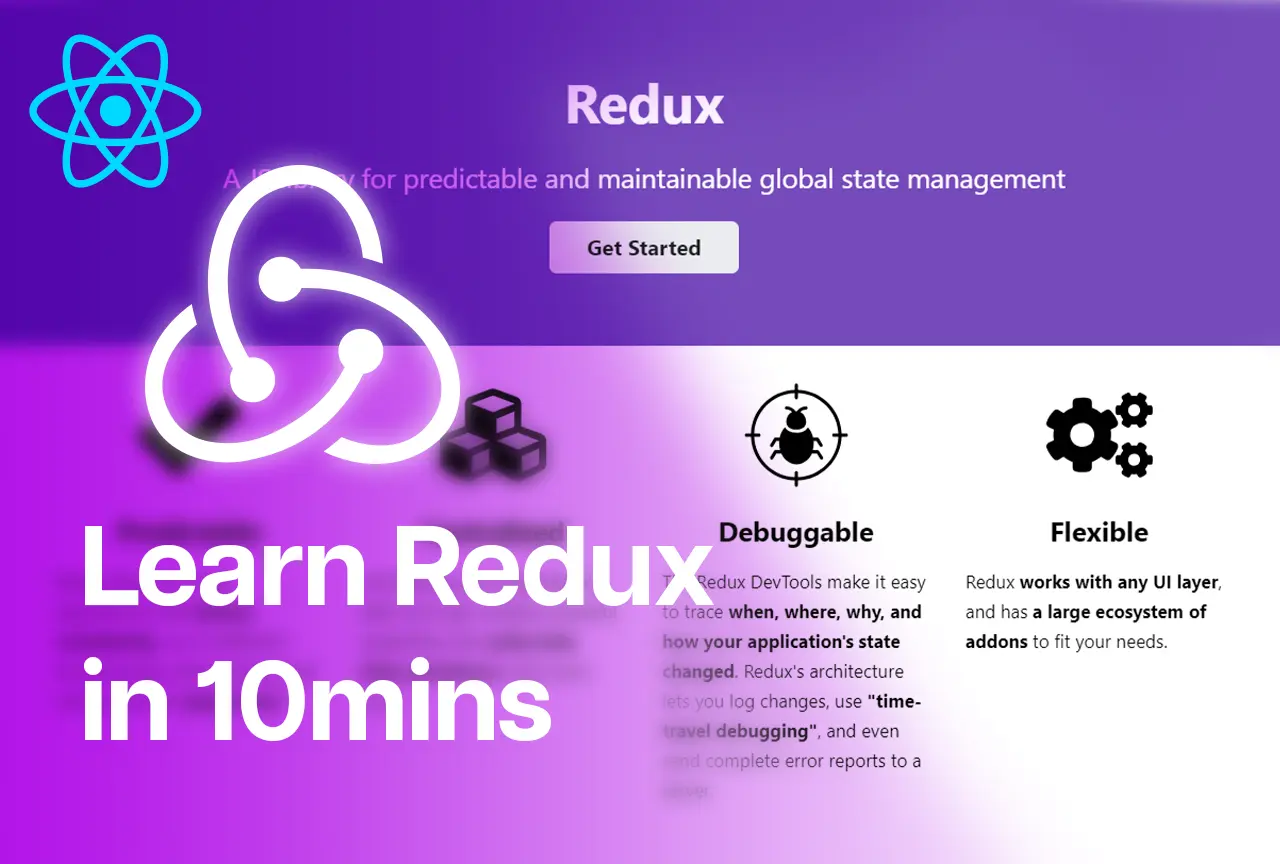
Table of Contents
Introduction to Redux
Redux is a state management library that provides a way to manage global state in JavaScript applications, particularly useful in React applications. It allows developers to manage application state in a predictable manner, making state accessible across components regardless of their location in the component tree. This guide will cover the core concepts of Redux, including the store, actions, and reducers, and will demonstrate how to effectively use Redux Toolkit to streamline your Redux development process.
The Core Concepts of Redux
Understanding the foundational concepts of Redux is crucial for effective state management. The three main concepts are:
- Store: The store holds the entire state of the application. It acts as a single source of truth that can be accessed from any component.
- Actions: Actions are plain objects that represent an intention to change the state. They must have a
type
property that indicates the type of action being performed. - Reducers: Reducers are pure functions that take the current state and an action as arguments and return a new state. They determine how the state changes in response to an action.
Setting Up Redux in Your Application
To set up Redux, you first need to create a store, define actions, and create reducers. Let’s walk through the process step by step using Redux Toolkit to simplify the setup.
Creating the Redux Store
To create a Redux store, you will need to use the configureStore
function from Redux Toolkit. This function simplifies the store setup process by automatically configuring the store with default middleware and enabling Redux DevTools integration.
import { configureStore } from '@reduxjs/toolkit';
export const store = configureStore({
reducer: {}
});
Defining State Slices
In Redux, the global state is often divided into slices, where each slice manages a specific part of the state. Using createSlice
from Redux Toolkit, you can easily define the initial state, reducers, and actions for each slice.
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
},
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
Connecting Redux to React
To connect Redux with a React application, you need to use the Provider
component from the React Redux library. This component makes the Redux store available to any nested components that need to access the Redux store.
import { Provider } from 'react-redux';
import { store } from './store';
function App() {
return (
);
}
Using Redux State and Dispatching Actions
Once Redux is set up, you can use the useSelector
hook to access the Redux state and the useDispatch
hook to dispatch actions from your components.
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './counterSlice';
function Counter() {
const count = useSelector((state) => state.counter.value);
const dispatch = useDispatch();
return (
<h2>{count}</h2>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>>Decrement</button>
);
}
Handling Asynchronous Actions
Redux Toolkit also provides utilities for handling asynchronous actions through createAsyncThunk
. This function allows you to create an action that performs an asynchronous operation and handles the different states (pending, fulfilled, and rejected) of the operation.
import { createAsyncThunk } from '@reduxjs/toolkit';
export const fetchCount = createAsyncThunk('counter/fetchCount', async (amount) => {
const response = await fetch(`/api/count?amount=${amount}`);
return response.data;
});
Debugging with Redux DevTools
Redux DevTools is an essential tool for debugging your Redux applications. It allows you to inspect every action and state change, making it easy to track down bugs and understand how state changes over time. To use Redux DevTools, you simply need to configure your store with the appropriate middleware.
const store = configureStore({
reducer: {},
devTools: process.env.NODE_ENV !== 'production',
});
Conclusion
Redux is a powerful state management library that, when used with Redux Toolkit, becomes much easier to implement and manage. By understanding the core concepts of Redux and leveraging the utilities provided by Redux Toolkit, you can efficiently manage global state in your React applications. This guide has covered the essential steps to get started with Redux and Redux Toolkit, enabling you to build scalable and maintainable applications.
Further Reading and Resources
Made with VideoToBlog